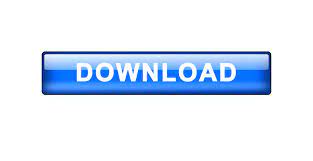
Try dumping the table to a text file, if you think there is stuff going on in there you cannot see. Row = row.replace(chr(10), eolchar).replace(chr(13), eolchar) With arcpy.da.UpdateCursor(tbl, ) as rows:
#Regular expression not eol code#
and the "with" construct helps you out by closing the cursor even if the code fails - avoiding the possibility of a nasty hanging file lock!ĭef strip_newlines(tbl, field, eolchar=""): I'm also using arcpy.da.UpdateCursor because it is very fast compared to the 10.0 flavor. as the usual use of "\n" breaks the geoprocessing messaging string representation in the code. Note I'm using chr(10) and chr(13) for "\n" and "\r" so this function can also be used inside the Calculate Value tool in modelbuilder. You could paste this function into the ArcMap python window and use it there. Here's a function implementation of your approach, not using hex codes. The regex house(cat|keeper) means match house followed by either cat or keeper.This method seems a little dangerous as I can envision where the string "0a" could occur in your hex string by accident, for example the two byte hex "b0 ac" represented this way would be an invalid match. Parts of a regex are grouped by enclosing them in parentheses. The grouping metacharacters () allow a part of a regex to be treated as a single unit. # Grouping things and hierarchical matching Here, all the alternatives match at the first string position, so the first matches. "cats" =~ /cats|cat|ca|c/ # matches "cats"Īt a given character position, the first alternative that allows the regex match to succeed will be the one that matches. "cats and dogs" =~ /dog|cat|bird/ # matches "cat"Įven though dog is the first alternative in the second regex, cat is able to match earlier in the string. Some examples: "cats and dogs" =~ /cat|dog|bird/ # matches "cat" If cat doesn't match either, then the match fails and Perl moves to the next position in the string. If dog doesn't match, Perl will then try the next alternative, cat. At each character position, Perl will first try to match the first alternative, dog. As before, Perl will try to match the regex at the earliest possible point in the string. To match dog or cat, we form the regex dog|cat. We can match different character strings with the alternation metacharacter '|'. "Hello World" =~ m /x # matches the whole string # Matching this or that If you're matching against $_, the $_ =~ part can be omitted: $_ = "Hello World" įinally, the // default delimiters for a match can be changed to arbitrary delimiters by putting an 'm' out front: "Hello World" =~ m!World! # matches, delimited by '!' Print "It matches\n" if "Hello World" =~ /$greeting/ The literal string in the regex can be replaced by a variable: $greeting = "World" The sense of the match can be reversed by using !~ operator: print "It doesn't match\n" if "Hello World" !~ /World/ This idea has several variations.Įxpressions like this are useful in conditionals: print "It matches\n" if "Hello World" =~ /World/ In our case, World matches the second word in "Hello World", so the expression is true. The operator =~ associates the string with the regex match and produces a true value if the regex matched, or false if the regex did not match. In this statement, World is a regex and the // enclosing /World/ tells Perl to search a string for a match. A regex consisting of a word matches any string that contains that word: "Hello World" =~ /World/ # matches The simplest regex is simply a word, or more generally, a string of characters. This page assumes you already know things, like what a "pattern" is, and the basic syntax of using them. This page covers the very basics of understanding, creating and using regular expressions ('regexes') in Perl. Perlrequick - Perl regular expressions quick start #DESCRIPTION
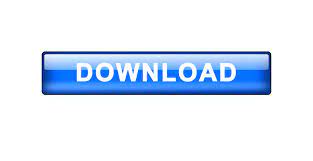